Troubleshooting C# cmd.exe Execution and Errors
This article explores the common issues and errors encountered during the execution of C# programs in cmd.exe, providing troubleshooting tips and solutions for a smoother development experience.
- Download and install the Exe and Dll File Repair Tool.
- The software will scan your system to identify issues with exe and dll files.
- The tool will then fix the identified issues, ensuring your system runs smoothly.
Introduction to c# cmd.exe
The C# cmd.exe is a command-line interface that allows users to execute commands and troubleshoot errors in their C# programs. To use cmd.exe, open the command prompt by typing “cmd” into the Windows search bar and pressing enter.
To execute a C# program, navigate to its directory using the “cd” command, then type “filename.exe” to run the program.
If you encounter errors during execution, it’s important to understand the error messages to identify and fix the issue. Common errors include missing or incorrect references, syntax errors, and runtime exceptions.
To troubleshoot errors, carefully review the error message and search for solutions online. Microsoft’s documentation and developer community forums are valuable resources for finding answers.
Purpose and Usage of c# cmd.exe
The purpose of the C# cmd.exe is to execute command-line instructions in the C# programming language. It is primarily used for troubleshooting and debugging C# programs. The cmd.exe is a command-line interface provided by Microsoft Windows operating system.
To use the C# cmd.exe, you need to open the command prompt and navigate to the directory where your C# program is located using the “cd” command. Once in the correct directory, you can execute your C# program by typing its filename followed by the “.exe” extension.
When troubleshooting C# cmd.exe execution and errors, it is important to understand the basics of the C# programming language and the syntax of the commands you are using. It is also helpful to have a good understanding of the directory structure and file management in Microsoft Windows.
If you encounter errors during execution, carefully review the error message displayed in the command prompt. This message often provides valuable information about the issue and can help you debug your C# program. Additionally, seeking support from the Microsoft community or referring to official documentation can provide further assistance in troubleshooting C# cmd.exe execution and errors.
Is c# cmd.exe Safe to Use?
C# cmd.exe is generally safe to use as long as proper precautions are taken. However, it is important to understand how it works and be mindful of potential errors that may occur. When troubleshooting C# cmd.exe execution and errors, there are a few key things to keep in mind.
Firstly, make sure you are familiar with the basics of using the cmd.exe command prompt. This includes understanding how to navigate directories, execute commands, and handle input and output.
If you encounter any errors while using C# cmd.exe, don’t panic. Take a systematic approach to troubleshooting by checking for common mistakes, such as typos in commands or incorrect file paths.
It is also helpful to refer to documentation and online resources for specific commands or error messages you encounter. The C# community is vast and there are numerous forums and websites where you can find solutions to common issues.
csharp
using System;
using System.Diagnostics;
class CommandLineTool
{
static void Main()
{
Console.WriteLine("Welcome to the Command Line Tool!");
while (true)
{
Console.Write("Enter a command (or 'exit' to quit): ");
string input = Console.ReadLine();
if (input.Equals("exit", StringComparison.OrdinalIgnoreCase))
break;
ExecuteCommand(input);
}
}
static void ExecuteCommand(string command)
{
ProcessStartInfo startInfo = new ProcessStartInfo
{
FileName = "cmd.exe",
Arguments = "/c " + command,
RedirectStandardOutput = true,
RedirectStandardError = true,
UseShellExecute = false,
CreateNoWindow = true
};
Process process = new Process();
process.StartInfo = startInfo;
process.OutputDataReceived += (sender, e) => Console.WriteLine(e.Data);
process.ErrorDataReceived += (sender, e) => Console.WriteLine(e.Data);
process.Start();
process.BeginOutputReadLine();
process.BeginErrorReadLine();
process.WaitForExit();
}
}
In this sample code, we have a simple command line tool that prompts the user to enter a command. It executes the command using `cmd.exe` and redirects the output and error streams to the console. The tool continues to prompt for commands until the user types “exit” to quit.
c# cmd.exe Legitimacy and Origin
C# cmd.exe is a legitimate command prompt utility in the C# programming language. It allows users to execute commands and perform various tasks on their computer.
To troubleshoot C# cmd.exe execution and errors, there are a few things you can try. First, double-check that the file you are trying to execute is in the correct directory. Use the cd command to navigate to the correct folder if needed.
If you are experiencing errors, make sure you are using the correct syntax for the command you are trying to execute. Check the documentation or search online for the correct syntax.
If you are encountering frequent errors, it may be helpful to run the cmd.exe as an administrator. Right-click on the cmd.exe icon and select “Run as administrator.”
Understanding c# cmd.exe as a System File
– C# cmd.exe is a system file that plays a crucial role in executing commands in the C# programming language.
– It is located in the “C:\Windows\System32” directory on a Windows computer.
– When troubleshooting C# cmd.exe execution and errors, it is important to ensure that the file is in the correct directory and not corrupted.
– To execute C# commands using cmd.exe, open the command prompt by pressing the “Windows + R” key and typing “cmd” in the Run dialog box.
– Use the cd command to navigate to the directory where the C# program is located.
– To compile a C# program, use the csc command followed by the name of the C# file.
– To run the compiled program, use the dotnet command followed by the name of the compiled file.
– If encountering errors, check for syntax errors in the C# code and ensure that all necessary libraries are referenced correctly.
– If needed, consult online resources or seek assistance from a programming community to troubleshoot specific C# cmd.exe errors.
Associated Software and Compatibility with c# cmd.exe
Associated Software | Compatibility with c# cmd.exe |
---|---|
Windows Command Prompt | Compatible |
PowerShell | Compatible |
Bash | Not compatible, requires additional setup |
Python | Compatible |
Latest Update: July 2025
We strongly recommend using this tool to resolve issues with your exe and dll files. This software not only identifies and fixes common exe and dll file errors but also protects your system from potential file corruption, malware attacks, and hardware failures. It optimizes your device for peak performance and prevents future issues:
- Download and Install the Exe and Dll File Repair Tool (Compatible with Windows 11/10, 8, 7, XP, Vista).
- Click Start Scan to identify the issues with exe and dll files.
- Click Repair All to fix all identified issues.
Exploring c# cmd.exe Creator and Download Options
To troubleshoot C# cmd.exe execution and errors, it’s important to have a clear understanding of the creator and download options available.
One option is to use the built-in C# cmd.exe creator, which allows you to create cmd.exe files directly from the C# programming language. This can be done by using the appropriate commands and syntax within your C# code.
Another option is to download pre-built cmd.exe files from reliable sources. These files are already created and can be downloaded and used directly without the need for additional coding.
When downloading cmd.exe files, it’s essential to choose reliable sources to ensure the safety and functionality of the files. Look for reputable websites and platforms that offer trusted cmd.exe downloads.
By exploring these creator and download options, you can effectively troubleshoot C# cmd.exe execution and errors, ensuring smooth and successful operations.
Troubleshooting c# cmd.exe Performance Issues
– Ensure that your computer meets the minimum system requirements for running C# cmd.exe. Check the processor, RAM, and storage capacity to ensure they are sufficient.
– Close any unnecessary background programs or processes that may be consuming system resources and impacting the performance of C# cmd.exe.
– Check for any errors or warnings in the event logs related to C# cmd.exe execution. This can help identify any underlying issues that may be causing performance problems.
– Utilize performance monitoring tools to track the resource usage of C# cmd.exe. This can help identify any spikes or excessive resource consumption that may be causing performance issues.
– Consider optimizing your code to improve the performance of C# cmd.exe. Look for areas where you can reduce computational complexity or improve algorithm efficiency.
– If the performance issues persist, try running C# cmd.exe in compatibility mode or as an administrator to see if that resolves the problem.
– Update your C# cmd.exe to the latest version to ensure you have access to any performance improvements or bug fixes.
Impact of c# cmd.exe on CPU Usage
The execution of C# cmd.exe commands can have a significant impact on CPU usage. When running commands, it is important to be aware of the potential strain it may put on your computer’s resources.
To troubleshoot any issues related to CPU usage, there are a few steps you can take. Firstly, check if any other processes or applications are running simultaneously that may be consuming a large amount of CPU power. Closing unnecessary programs can help alleviate the strain.
Another factor to consider is the complexity and efficiency of the commands being executed. Writing efficient code and optimizing your commands can reduce CPU usage.
Additionally, monitoring the CPU usage during the execution can provide insights into any potential bottlenecks or issues. Use the task manager or a performance monitoring tool to keep an eye on the CPU usage.
Running c# cmd.exe in the Background
To run a C# cmd.exe in the background, follow these steps:
1. Open your C# program and import the necessary namespaces.
2. Use the Process class to start the cmd.exe process.
3. Set the WindowStyle property of the process to Hidden.
4. Specify the UseShellExecute property as false to run the command directly.
5. Set the CreateNoWindow property to true to prevent the command window from appearing.
6. Specify the command to be executed using the StartInfo property of the process.
7. Use the Start method to start the process.
8. Check for any errors or exceptions that may occur during the execution.
By running the cmd.exe in the background, you can troubleshoot and resolve any issues with your C# program without the command window interrupting your workflow.
Dealing with Unresponsiveness of c# cmd.exe
If you’re experiencing unresponsiveness with the C# cmd.exe, there are a few troubleshooting steps you can take.
First, try checking your computer’s resources to ensure there is enough memory and processing power available. If your system is overloaded, it can cause the cmd.exe to become unresponsive.
Next, verify that your command syntax is correct. Double-check the command you are trying to execute and ensure it is properly formatted.
If the issue persists, try running cmd.exe as an administrator. This can help resolve any permission-related problems that may be causing the unresponsiveness.
You may also want to scan your computer for malware. Malicious software can interfere with the proper execution of cmd.exe, so it’s important to rule out any potential threats.
If all else fails, consider reinstalling C# cmd.exe. This can help resolve any corrupted files or settings that may be causing the issue.
c# cmd.exe Malware and Removal Tools
- Open Task Manager by pressing Ctrl+Shift+Esc.
- Click on the Startup tab.
- Look for any suspicious entries related to c# cmd.exe.
- Select the suspicious entry and click on Disable.
- Restart your computer.
Repair Method 2: Scan for Malware
- Download and install a reputable anti-malware software.
- Launch the anti-malware software.
- Perform a full system scan.
- If any malware is detected, follow the software’s instructions to remove it.
- Restart your computer.
Repair Method 3: Use System Restore
- Press Windows Key + R to open the Run dialog box.
- Type rstrui and press Enter.
- In the System Restore window, click on Next.
- Select a restore point before the issue started occurring.
- Follow the on-screen instructions to restore your system.
- Restart your computer.
Repair Method 4: Reinstall C# and cmd.exe
- Open Control Panel.
- Click on Programs or Programs and Features.
- Find C# in the list of installed programs.
- Click on Uninstall or Change.
- Follow the on-screen instructions to uninstall C#.
- Restart your computer.
- Download the latest version of C# from the official website.
- Install C# by following the installation instructions.
- Repeat steps 1-5 for cmd.exe.
- Restart your computer.
Exploring c# cmd.exe Startup and Updates
When troubleshooting C# cmd.exe execution and errors, it is important to understand the startup process and how to handle updates. To start, make sure the computer file for cmd.exe is located in the correct directory. Then, open the cmd.exe command prompt by pressing the Windows key + R and typing “cmd” before hitting Enter.
If you encounter errors during startup, try running cmd.exe as an administrator by right-clicking the cmd.exe icon and selecting “Run as administrator.” This can help resolve permission-related issues.
To check for updates, use the ver command in the command prompt to display the version of cmd.exe. If you need to update cmd.exe, visit the Microsoft website to download the latest version.
Alternatives to c# cmd.exe
If you are experiencing issues with the c# cmd.exe execution or encountering errors, there are alternatives you can try.
One option is to use PowerShell, a powerful command-line tool that offers more functionality and flexibility than cmd.exe. PowerShell allows you to automate tasks and manage system configurations.
Another alternative is the Windows Terminal, a modern command-line application that supports multiple command-line tools, including PowerShell and cmd.exe. It provides a more customizable and user-friendly interface.
If you prefer a graphical user interface (GUI) over the command-line, you can use Visual Studio, an integrated development environment (IDE) for C# development. Visual Studio allows you to write, debug, and execute C# code with ease.
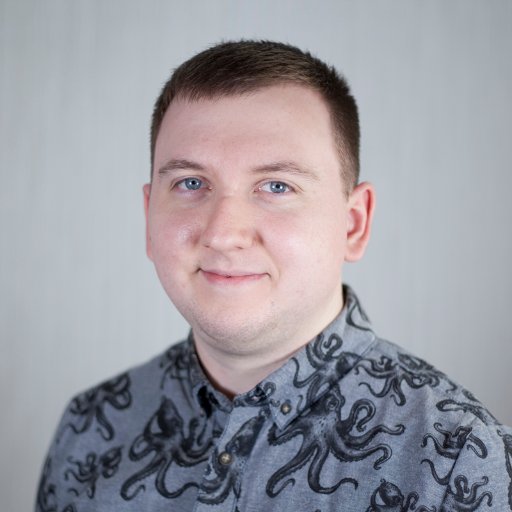