Executing EXE with Parameters in PowerShell
In this article, we will explore the process of executing EXE files with parameters using PowerShell.
- Download and install the Exe and Dll File Repair Tool.
- The software will scan your system to identify issues with exe and dll files.
- The tool will then fix the identified issues, ensuring your system runs smoothly.
Purpose of PowerShell execute exe with parameters function
The PowerShell execute exe with parameters function allows you to run an executable file (.exe) with specific arguments or parameters from a PowerShell script or command line. This is especially useful when you want to automate tasks or interact with other programs.
To execute an exe with parameters in PowerShell, you can use the Start-Process cmdlet. This cmdlet allows you to specify the path to the exe file and the arguments you want to pass to it. For example:
Start-Process -FilePath “C:\Program Files\MyApp.exe” -ArgumentList “/arg1 value1 /arg2 value2”
In this example, “C:\Program Files\MyApp.exe” is the path to the executable file, and “/arg1 value1 /arg2 value2” are the arguments or parameters you want to pass to the exe.
By using the Start-Process cmdlet, you can easily execute exe files with parameters in PowerShell and automate various tasks.
Origin and associated software of PowerShell execute exe with parameters
When using PowerShell to execute an EXE file with parameters, there are a few things to keep in mind. First, make sure you have the correct command syntax. Effective PowerShell commands utilize the “Start-Process” cmdlet followed by the path to the EXE file. After that, you can add the necessary arguments using the “-ArgumentList” parameter.
If your parameters contain spaces or special characters, be sure to wrap them in quotes. Additionally, if you need to pass multiple parameter/argument pairs, you can use a string with the format “Param1=Value1 Param2=Value2”.
For example, if you wanted to run the “XendesktopServerSetup.exe” file located in “C:\X64\Xendesktop Setup”, you would use the following command:
Start-Process -FilePath “C:\X64\Xendesktop Setup\XenDesktopServerSetup.exe” -ArgumentList “/CONFIGURE_FIREWALL /NOSQL”
This command will execute the EXE file with the specified parameters.
Legitimacy and safety of executing exe files with parameters in PowerShell
Executing EXE files with parameters in PowerShell is a legitimate and safe practice when done correctly. To execute an EXE file with parameters in PowerShell, you can use the “Start-Process” cmdlet and specify the path to the executable file, as well as the desired parameters.
For example, if you want to execute an EXE file called “XenDesktopServerSetup.exe” located in the “X64\Xendesktop Setup” folder, you can use the following command:
Start-Process -FilePath “X64\Xendesktop Setup\XenDesktopServerSetup.exe” -ArgumentList “Arg1”, “Arg2”, “Arg3”
Make sure to enclose the file path and arguments in quotes if they contain spaces or special characters. This ensures that PowerShell interprets them correctly.
When executing EXE files with parameters, it is important to consider security. Always run PowerShell scripts with the appropriate permissions, such as running as an administrator. Additionally, be cautious when executing EXE files obtained from untrusted sources and ensure that they come from reputable sources.
powershell
$exePath = "C:\Path\to\your\executable.exe"
$param1 = "parameter1"
$param2 = "parameter2"
Start-Process -FilePath $exePath -ArgumentList $param1, $param2 -Wait
In the above code, we first define the path to the executable file (`$exePath`) and the parameters that need to be passed to it (`$param1` and `$param2`). The `Start-Process` cmdlet is then used to execute the executable file, passing the parameters using the `-ArgumentList` parameter. The `-Wait` flag is used to make PowerShell wait for the executable to finish before proceeding.
Please remember to update the `$exePath`, `$param1`, and `$param2` variables with the appropriate values according to your specific use case.
Let me know if you have any further questions or if there’s anything else I can assist you with!
Usage and benefits of PowerShell execute exe with parameters
PowerShell’s ability to execute executable files with parameters offers numerous benefits.
By using the Start-Process cmdlet, you can easily run an executable file with specific arguments, providing a more efficient and automated way to perform tasks. This is especially useful when working with complex or repetitive tasks.
For example, you can use PowerShell to deploy applications or tools like msdeploy.exe to specific locations, saving time and effort. You can also use it to schedule tasks using the Task Scheduler, ensuring that they run smoothly and without manual intervention.
Additionally, PowerShell’s ability to execute exe files with parameters allows for silent installations, eliminating the need for user interaction. This can be achieved by using the Start-Process cmdlet with the appropriate arguments.
Malware concerns and potential risks of PowerShell execute exe with parameters
Malware concerns arise when executing EXE files with parameters in PowerShell. It is important to be aware of the potential risks and take necessary precautions to ensure security.
To mitigate these risks, follow these steps:
1. Only run executable files from trusted sources and verify their authenticity.
2. Use a secure account with limited privileges when executing PowerShell commands.
3. Keep your IT peers informed about the process and seek their advice if needed.
4. Familiarize yourself with PowerShell’s security features and best practices.
5. Consider using a reputable antivirus software to scan the EXE files before execution.
6. Be cautious of any suspicious or unexpected behavior during the execution process.
7. Regularly update and patch your operating system and PowerShell to prevent vulnerabilities.
Troubleshooting and resolving issues with PowerShell execute exe with parameters
Troubleshooting and resolving issues with executing EXE files with parameters in PowerShell can be a daunting task, but with the right guidance, it can be easily resolved.
To start, make sure you are running PowerShell as an administrator to avoid any permission issues. Once in PowerShell, use the “Start-Process” cmdlet to execute the EXE file. Make sure to include the path of the EXE file and any necessary parameters.
If you are unsure about the correct parameters to use, you can use the “echoargs” utility to display the arguments that are passed to the EXE file. This can help you identify any issues with the arguments you are using.
If you are encountering issues with spaces in your arguments, make sure to enclose the argument in double quotes or use the backtick (`) character to escape the space.
If you need to schedule the execution of the EXE file, you can use the Windows Task Scheduler to automate the process.
Latest Update: July 2025
We strongly recommend using this tool to resolve issues with your exe and dll files. This software not only identifies and fixes common exe and dll file errors but also protects your system from potential file corruption, malware attacks, and hardware failures. It optimizes your device for peak performance and prevents future issues:
- Download and Install the Exe and Dll File Repair Tool (Compatible with Windows 11/10, 8, 7, XP, Vista).
- Click Start Scan to identify the issues with exe and dll files.
- Click Repair All to fix all identified issues.
High CPU usage and performance impact of running exe files with parameters in PowerShell
When running an exe file with parameters in PowerShell, it is important to be aware of the potential impact on CPU usage and performance.
To ensure efficient execution, consider the following tips:
1. Use the appropriate command: Instead of using a batch file or cmd.exe, utilize PowerShell’s Start-Process cmdlet to run the exe file with parameters. This provides more control and flexibility.
2. Optimize parameter handling: Use the Args property to pass parameters to the exe file. Splitting parameters using the Split method can be useful for complex parameter strings.
3. Minimize unnecessary processes: Avoid running multiple instances of PowerShell or other unnecessary processes simultaneously, as this can lead to high CPU usage and performance degradation.
4. Run as administrator: Certain exe files may require administrative privileges. To ensure smooth execution, run the PowerShell script or batch file as an administrator.
System file implications and impact on Windows versions compatibility
When executing an EXE with parameters in PowerShell, it is important to consider the system file implications and the impact on Windows versions compatibility.
To effectively run an EXE with parameters in PowerShell, the “Start-Process” cmdlet is commonly used. This allows you to specify the EXE file, any necessary arguments or switches, and the desired working directory.
For example, to run an EXE called “Deploy\msdeploy.exe” with parameters “Prms” and “Parms.Split(‘ ‘)”, you can use the following command:
Start-Process -FilePath "Deploy\msdeploy.exe" -ArgumentList "Prms", "Parms.Split(' ')" -WorkingDirectory "DESKTOPSTUDIO"
It is important to ensure that the PowerShell script is run with the necessary permissions, so it may be necessary to run PowerShell as an administrator.
By using PowerShell to execute EXE files with parameters, you can automate tasks, perform silent installations, and pass command line arguments easily. This is a powerful solution for system administrators and IT professionals seeking to streamline their workflows.
Process description and behavior of PowerShell execute exe with parameters
When using PowerShell to execute an EXE with parameters, there are a few key steps to follow. First, make sure you have a clear understanding of the arguments you need to pass to the EXE. Use echoargs or echoargs -verb to display the arguments in the PowerShell console. Next, use the Start-Process cmdlet to run the EXE.
Specify the path to the EXE file and use the -ArgumentList parameter to pass the arguments. If the EXE requires administrative privileges, run PowerShell as an administrator. You can also call the EXE from a batch file using the cmd. exe command.
Ending task and safe termination of PowerShell execute exe with parameters
To safely terminate a PowerShell execution of an executable with parameters, follow these steps:
1. Open Windows PowerShell as an administrator.
2. Use the “Start-Process” cmdlet to execute the EXE file with its required parameters. For example: Start-Process -FilePath “C:\Path\to\your\file.exe” -ArgumentList “Arg1”.
3. If needed, specify additional switches or arguments for the execution.
4. To end the task, press “Ctrl+C” on your keyboard or close the PowerShell window.
Steps to remove or delete PowerShell execute exe with parameters
1. Open PowerShell as an administrator.
2. Use the Remove-Item cmdlet to delete the PowerShell execute exe file. Specify the file’s location and name.
3. If the file is currently in use, you may need to stop any processes or services that are using it before deleting.
4. If you’re unsure about the file’s location, you can use the Get-Location cmdlet to get the current directory.
5. To delete multiple files at once, you can use wildcards in the file name or path.
6. After deleting the file, you can confirm its removal by using the Test-Path cmdlet.
7. If you encounter any errors during the deletion process, you can use the -Force parameter with the Remove-Item cmdlet to forcibly remove the file.
Recommended removal tools and alternative methods for executing exe files in PowerShell
Recommended Removal Tools and Alternative Methods for Executing EXE Files in PowerShell
When it comes to executing EXE files with parameters in PowerShell, there are a few recommended removal tools and alternative methods that can be helpful. One effective PowerShell command for running an EXE file with arguments is the “Start-Process” command. This command allows you to specify the path to the EXE file and any necessary arguments.
An alternative method is to use the call operator, indicated by the “&” symbol, followed by the path to the EXE file and its arguments. This method can be useful when you want to run batch files or bat files silently.
If you’re looking for a more visual solution, you can also use the “psExec” tool developed by Mark Russinovich. This tool allows you to execute EXE files with parameters from the command line or from a script.
It’s important to note that when executing EXE files with PowerShell, it’s recommended to run the PowerShell session as an administrator. This ensures that the necessary permissions are in place to successfully execute the file.
By utilizing these recommended removal tools and alternative methods, you can effectively execute EXE files with parameters in PowerShell and accomplish your tasks more efficiently.
Background execution and impact on system startup
Background execution in PowerShell refers to the ability to run an EXE file with parameters without the need for user interaction. This feature has a significant impact on system startup as it allows for the automation of tasks during the boot process.
To execute an EXE with parameters in PowerShell, you can use the “Start-Process” cmdlet. This cmdlet allows you to specify the path to the EXE file and provide any necessary arguments or parameters. For example, if you want to run an EXE file called “example.exe” with the argument “Arg 1”, you can use the following command:
Start-Process -FilePath "C:\Path\to\example.exe" -ArgumentList "Arg 1"
By running this command, the EXE file will be executed in the background, without any user interface or prompts. This is especially useful for tasks that need to be performed during system startup, such as configuring the firewall or performing silent installations.
Background execution in PowerShell is a valuable tool for IT professionals and PowerShell experts who need to automate tasks and streamline system startup. It allows for efficient and seamless execution of EXE files with parameters, saving time and effort for both the person executing the command and their IT peers.
By utilizing background execution in PowerShell, tasks can be performed silently and efficiently, ensuring a smooth system startup process.
Updates and downloads related to PowerShell execute exe with parameters
- PowerShell version compatibility: Ensure that your PowerShell version is compatible with executing EXE files with parameters.
- Download the required EXE: Locate and download the EXE file that you want to execute with parameters. Ensure that it is the correct version and compatible with your system.
- Identify the parameters: Understand the parameters required by the EXE file. Refer to the documentation or contact the software vendor for information on the available parameters.
- Open PowerShell: Launch the PowerShell application on your computer.
- Set the execution policy: If needed, set the PowerShell execution policy to allow running scripts or EXE files. Use the Set-ExecutionPolicy cmdlet to configure the policy.
- Navigate to the directory: Use the
cd
command to navigate to the directory where the EXE file is located. - Execute the EXE with parameters: Use the
Start-Process
cmdlet to execute the EXE file with the specified parameters. Provide the path to the EXE file and the required parameters as arguments. - Verify the execution: Confirm that the EXE file executed successfully and that the desired actions or operations were performed.
- Further troubleshooting: If you encounter any issues or errors, refer to the PowerShell documentation or seek assistance from the software vendor or community forums.
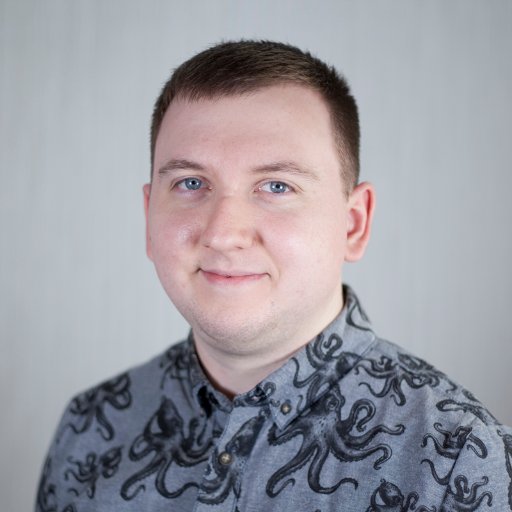